前些天跟同学一起糊实验室的项目,要做个实验平台,想实现一个点击实验步骤然后块的内容就会变成对应步骤的功能,当时糊了一个页内链接,贼丑,然后同学用js控制了内容的显隐实现了这个功能,今天看到arxiv上有类似的块,查了点资料,学习一下实现方法
总的来说是要实现这样一个东西
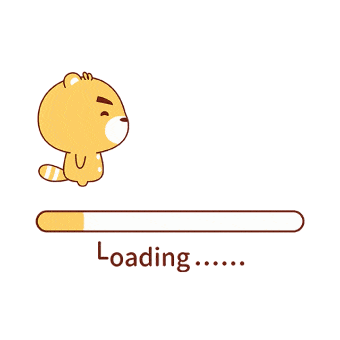
点击上面的lable然后就可以切换下面的内容框内的文字
单选按钮radio
首先需要用到的是input标签中的单选按钮radio
下面给出一个示例
1 2 3 4 5
| <div class="tab"> <input type="radio" name="tab-group-1">选项一 <input type="radio" name="tab-group-1">选项二 <input type="radio" name="tab-group-1">选项三 </div>
|
效果如下
选项一
选项二
选项三
注意我们需要给每个input标签一个相同的name表示他们属于同一组radio,这样子才能起到单选的效果
绑定lable与radio
现在我们想要给每个选项外边套一个框,这个可以通过绑定lable和radio来实现
具体我们来看代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <style> .tab label { background: #eee; padding: 10px; border: 1px solid #ccc; position: relative; } </style> <div class="tab"> <input type="radio" name="tab-group-1" id="tab-1"> <label for="tab-1">选项一</label> <input type="radio" name="tab-group-1" id="tab-2"> <label for="tab-2">选项二</label> <input type="radio" name="tab-group-1" id="tab-3"> <label for="tab-3">选项三</label> </div>
|
实现效果如下
绑定用的是label标签的for属性,通过id和radio绑定,绑定的效果就是当你点击label的时候,起到点击input同样的效果
这里我们发现前面那个圆点显然是我们不需要的,所以我们在css中把它隐藏掉
1 2 3
| .tab [type=radio] { display: none; }
|
就可以得到这样的效果
radio的checked效果
现在我们看到这三个label没啥区别,我们希望我们选中label的时候能够有变化,比如边框底部的线消失,颜色变化 (先不考虑下面内容框的显示)
这里我们需要给选中状态check额外添加一个css属性
1 2 3 4
| .tab [type=radio]:checked ~ label { background: white; border-bottom: 1px solid white; }
|
这里~
符号表示的是同一个父元素下该元素后的所有元素
所以这里是tab的radio后所有的label,所以这里我们就不能将所有label放在一个tab里面了,要分多个tab来放,同时对tab设置一个float: left
效果如下
z-index的使用
最后我们来加上内容框,首先设置一个内容框的css,用tabs把所有东西框起来
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| .content { position: absolute; top: 30px; left: 0; background: white; right: 0; bottom: 0; padding: 20px; border: 1px solid #ccc; } .tabs { position: relative; min-height: 200px; clear: both; margin: 25px 0; }
|
然后我们把内容框加到html代码中去
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <div class="tabs"> <div class="tab"> <input type="radio" id="tab-1" name="tab-group-1"> <label for="tab-1">选项一</label> <div class="content"> 内容一 </div> </div> <div class="tab"> <input type="radio" id="tab-2" name="tab-group-1"> <label for="tab-2">选项二</label> <div class="content"> 内容二 </div> </div> <div class="tab"> <input type="radio" id="tab-3" name="tab-group-1"> <label for="tab-3">选项三</label> <div class="content"> 内容三 </div> </div> </div>
|
我们发现最后一个内容框会把前两个内容框盖掉,并且之前的下边框横线消失也会被内容框盖住
这里需要用到css中一个属性叫z-index,这个属性可以规定当元素重叠时哪个元素盖在上面 (值大的盖在上面),有了这个东西,我们将checked,也就是选中的元素放在最上面,不就实现了切换的功能么
1 2 3 4 5 6
| [type=radio]:checked ~ label { z-index: 2; } [type=radio]:checked ~ label ~ .content { z-index: 1; }
|
然后,在input属性中加入checked用于标记默认显示,这个标签页功能就实现了
代码总览
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| .tabs { position: relative; min-height: 200px; clear: both; margin: 25px 0; } .tab { float: left; } .tab label { background: #eee; padding: 10px; border: 1px solid #ccc; position: relative; } .tab [type=radio] { display: none; } .content { position: absolute; top: 30px; left: 0; background: white; right: 0; bottom: 0; padding: 20px; border: 1px solid #ccc; } .tab [type=radio]:checked ~ label { background: white; border-bottom: 1px solid white; z-index: 2; } .tab [type=radio]:checked ~ label ~ .content { z-index: 1; }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <div class="tabs"> <div class="tab"> <input type="radio" id="tab-1" name="tab-group-1" checked> <label for="tab-1">选项一</label> <div class="content"> 内容一 </div> </div> <div class="tab"> <input type="radio" id="tab-2" name="tab-group-1"> <label for="tab-2">选项二</label> <div class="content"> 内容二 </div> </div> <div class="tab"> <input type="radio" id="tab-3" name="tab-group-1"> <label for="tab-3">选项三</label> <div class="content"> 内容三 </div> </div> </div>
|